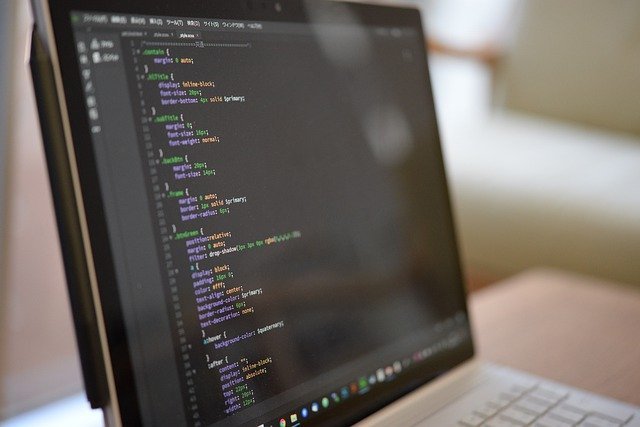
The majority of styles that are applied to Material-UI components are part of the theme styles. In some cases, you need the ability to style individual components without changing the theme. For example, a button in one feature might need a specific style applied to it that shouldn’t change every other button in the app. Material-UI provides several ways to apply custom styles to components as a whole, or to specific parts of components.
This article is taken from the book React Material-UI Cookbook by Adam Boduch by Adam Boduch. This book will serve as your ultimate guide to building compelling user interfaces with React and Material Design. Filled with practical and to-the-point recipes, you will learn how to implement sophisticated-UI components. To follow along with the examples implemented in this article, you can download the code from the book’s GitHub repository.
In this article, we will look at the various styling solutions to design appealing user interfaces including basic component styles, scoped component styles, extending component styles, moving styles to themes, and others.
Basic component styles
Material uses JavaScript Style Sheets (JSS) to style its components. You can apply your own JSS using the utilities provided by Material-UI.
How to do it…
The withStyles() function is a higher-order function that takes a style object as an argument. The function that it returns takes the component to style as an argument. Here’s an example:
import React, { useState } from 'react';import { withStyles } from '@material-ui/core/styles';import Card from '@material-ui/core/Card';import CardActions from '@material-ui/core/CardActions';import CardContent from '@material-ui/core/CardContent';import Button from '@material-ui/core/Button';import Typography from '@material-ui/core/Typography';const styles = theme => ({card: {width: 135,height: 135,textAlign: 'center'},cardActions: {justifyContent: 'center'}});const BasicComponentStyles = withStyles(styles)(({ classes }) => {const [count, setCount] = useState(0);const onIncrement = () => {setCount(count + 1);};return (<Card className={classes.card}><CardContent><Typography variant="h2">{count}</Typography></CardContent><CardActions className={classes.cardActions}><Button size="small" onClick={onIncrement}>Increment</Button></CardActions></Card>);});export default BasicComponentStyles;
Here’s what this component looks like:
How it works…
Let’s take a closer look at the styles defined by this example:
const styles = theme => ({ card: { width: 135, height: 135, textAlign: 'center' }, cardActions: { justifyContent: 'center' } });
The styles that you pass to withStyles() …….
Source: https://hub.packtpub.com/applying-styles-to-material-ui-components-in-react-tutorial/